Program to Find Path With the Minimum Effort in Python
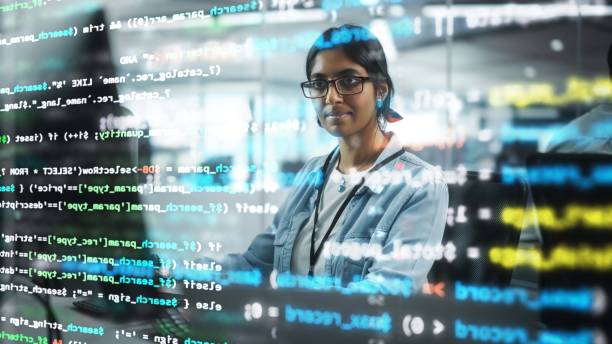
When you step inside the coding universe, you will encounter some of the most interesting topics! From arrays and subarrays to binary trees to reverse words in a string, you come across several important topics. One such coding topic that is asked from the aspirants is path with minimum effort in python. Here, you need to find a route that you wish to take. Here, this route will be found with minimum effort.
Dive deep inside this topic and know how to find the path with minimum effort in detail!
Let’s get started!
Consider the problem statement
You are having ninja training for the upcoming battle. A height, a given 2d array for rows and columns with height [ rows] [col] representing the needed height of a given cell is present.
Suppose you are standing at a top-left cell i.e [0,0]. You need to travel from its bottom-right cell [ which is row 1 and column 1]. You can easily move left, right or up and down and may also need to find a route that may require a minimum path.
The route time is considered as the absolute differences in the height between any two given consecutive cells of the required route. Then return the required minimum time to travel in case of its top-left cell to its bottom-left cell.
Input would be: Height [ 1, 2, 2] [ 3, 8, 2 ] [ 5, 3, 5 ]
The output in this case would be 2.
The route for the [ 1, 3, 5, 3, 5] will be having the needed maximum absolute differences as 3.
Input format:
Here, in the first line you can determine the required test cases in a problem. Each test case’s first line will contain two spaces that are separated by the integers having the required value in the first row. Second, would be the number of required columns in a 2d array.
Now in a 2d array, the input would be taken as the rows or columns that are entered there before. Also, lines in the input will be equal to the required number of rows while they would be separated by certain integers for each given row.
For example; the given row is 3 and the column is also 3. We will be having the 3 lines of input that are separated by the integers.
Output format:
For all the test cases, you can return the minimum time that is required in order to travel from a top-left cell to its bottom-left cell. You can print the desired output in some other line.
Approaches to follow
In order to find the path with the minimum efforts, you can follow below-mentioned approaches.
Approach 1
The basic idea behind this approach would be to use the dfs method in order to implement the parameter of TIME_LIMIT. It will be the maximum time that is needed to deal.
The idea here would be to use the binary search tree in order to find the small TIME_LIMIT to reach at the end point.
Algorithm:
- Ideally initialise the length of all rows, columns and the neighbours in a 2d list that consists of several moves that needs to performed on the height list
- Define the function as TIME_LIMIT for the maximum time to reach a particular destination. Take x and y coordinates for each of the iterations. Perform DFS traversal to visit all the neighbour nodes but make sure to not go out of a grid. Take into consideration the cells which are not yet visited
- You can also make use of a binary search tree. Though, if the TIME_LIMIT = -1, you may not reach at the end.
- Return the minimum time that is required to traverse from the source till its destination.
Approach 2
The main idea of this approach would be to think how a program will be modelled with the help of an undirected graph and can be solved with the help of a Dijkstra algorithm with the help of BFS search traversal.
Algorithm:
- First initialise the rows, columns, 2d lists or arrays. The heights of the given 3d cells in this case are initialised with the neighbouring array that comprises of all valid set of moves
- Initialize required priority_queue which needs to get the time of cell [i,j]
- Traverse all the items that are present in any queue until that queue will become empty. Take the note of minimum time which is required to reach the node
- Extract all cells with minimum time in order to look at all its neighbours
- For all iterations, you can pop vertex with that of the minimum time.
- Though, if the time is short than its current best time, relax the value to take it to the heap
- End the required loop to reach at the bottom cell and to return the value of the minimum time that you have obtained
Program solution
Consider the program solution to find the path with the minimum efforts:
Class solution:
def minimumeffortPath (Self, heights:
List [ list [ int]] – > int:
Start = 0
end = 1000000
while start + 1 < end:
Mid = [ start + end ] // 2
if self.dfs [ heights, set (), 0, 0 , mid]:
end = mid
Else:
start = mid
if self.dfs [ heights, set (), 0, 0, mid):
end = mid
Else:
start = mid
If self.dfs (height, set (), 0, 0, start):
Return start
If self.dfs (height, set (), 0, 0, end)
Return end
return-1
def dfs [ self, height, visited, x, y, limit):
If x = len[heights] – 1 and y = lens [heights [0] -1:
return true
The time complexity in this case would be the O [MN] where the M signifies the number of rows in a given matrix and the N signifies the number of columns.
The space complexity in this case will be O [MN]
Wrapping Up
From arrays to binary heap to reversing words in a string, you come across several interesting coding topics.
In this blog, we have tried to explain in-depth the topic of path with minimum effort. Read this blog and keep it as your benchmark to ace on it.